プログラミングシリーズの続きとして、関数について説明します。これには、関数(Function)に伴うすべてのことの多くが含まれます。コーディング方法を学びたい場合は、関数を理解することが非常に重要です。同じことが、仕事の別のセクションで使用するためにコードを頻繁にコピーする傾向がある現在のプログラマーにも当てはまります。
関数の使い方を学ぶことは、コーダーがより効率的に作業する方法を知っていることを意味します。それだけでなく、コードが読みやすくなり、チームで作業している場合はそれが恩恵になります。
プログラミングの機能とは何ですか?
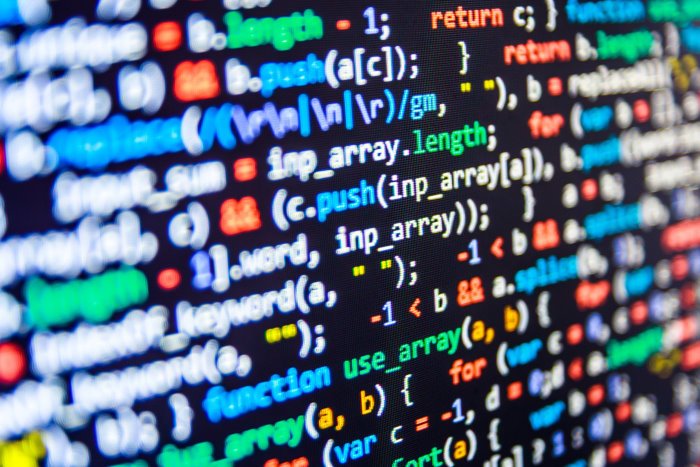
基本的に、関数はさまざまなタスクを実行するコードのブロックです。必要に応じて、関数を何度も呼び出して再利用できます。さらに面白くするために、コーダーは情報を関数に簡単に渡すことができますが、それだけでなく、情報をすぐに送り返すこともできます。
現時点では、人気のあるプログラミング言語の多くにこの機能が組み込まれています。これは現時点で期待されています。
これで、関数が呼び出されるたびに、プログラムは通常、現在実行中のプログラムを一時停止し、関数を実装します。そこから、関数は上から下に読み取られ、関数がタスクを完了すると、プログラムは一時停止したところから続行します。
関数が値を送り返す場合、その特定の値は、関数が最初に呼び出された場所で使用されます。
読む(Read):Javaプログラミング言語とは何(What is Java Programming language)ですか?
ボイド関数の書き方
OK、void関数の記述は非常に簡単で、短時間で実行できます。この関数は値を返さ(Bear)ないことに注意してください。あなたに何をすべきかについての考えを与えるかもしれないいくつかの例を見てみましょう。
JavaScriptの例
function helloFunction(){
alert("Hello World!");
}
helloFunction();
Pythonの例
def helloFunction():
print("Hello World")
helloFunction()
C++の例
#include <iostream>
using namespace std;
void helloFunction(){
cout << "Hello World!";
}
int main(){
helloFunction();
return 0;
}
読む(Read): Rプログラミング言語とは何(What is the R programming language)ですか?
値を必要とする関数の書き方
作業中に同じコードを数回記述している場合は、void関数が最適です。ただし、これらのタイプの関数は変更されないため、非常に便利ではありません。void関数をより有益にする最良の方法は、関数に異なる値を送信することによって、それらが実行できることを増やすことです。
Pythonの例
def helloFunction(newPhrase):
print(newPhrase)
helloFunction("Our new phrase")
JavaScriptの例
function helloFunction(newPhrase){
alert(newPhrase);
}
helloFunction("Our new phrase");
C++の例
#include <iostream>
using namespace std;
void helloFunction(string newPhrase){
cout << newPhrase;
}
int main(){
helloFunction("Our new Phrase");
return 0;
}
読む(Read):すべてのプログラマーが従うべき最良のプログラミング原則とガイドライン(Best Programming Principles & Guidelines all Programmers should follow)。
値を返す関数の書き方
したがって、この記事の最後の側面は、値を返す関数を作成する方法です。データを使用する前にデータを変更したい場合は、これがほとんどの状況での方法です。
Pythonの例
def addingFunction(a, b):
return a + b
print(addingFunction(2, 4))
JavaScriptの例
function addingFunction(a, b){
return a + b;
}
alert(addingFunction(2, 4));
C++の例
#include <iostream>
using namespace std;
int addingFunction(int a, int b){
return a + b;
}
int main(){
cout << addingFunction(2, 4) ;
return 0;
}
読む(Read):初心者プログラマーのための最高のプロジェクト(The best projects for beginner Programmers)。
(Have)ここにリストしたコードを楽しんでテストしてください。それらがあなたの仕事に役立つことを願っています。
What is a Function in Programming? We explain
In continuation with our programming series, we are going to talk about Function – much of all that it entails. If you want to learn how to code, then understanding functions is really important. The same applies to current programmers who tend to copy their code quite often to use in a different section of their work.
Learning how to use functions means the coder will know how to work more efficiently. Not only that, but the code will be easier to read, and that is a boon if you’re working in a team.
What is a Function in Programming?
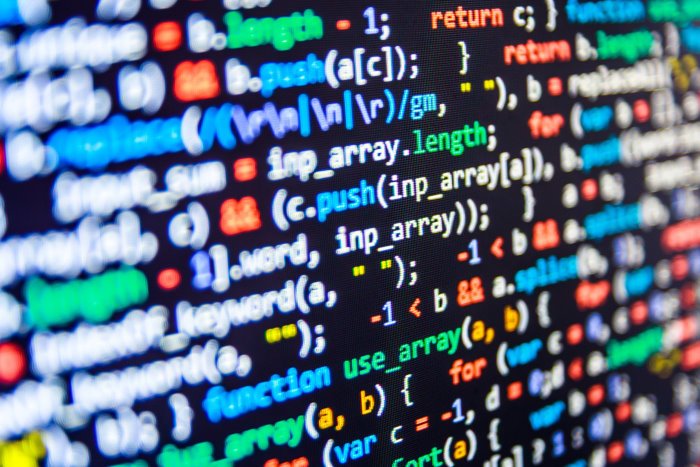
In basic terms, a function is a block of code that performs various tasks. Should you need to, a function can be called and reused numerous times. To make things even more interesting, coders can pass information to a function with ease, but not only that, but it is also possible to send information right back.
At the moment, many of the popular programming languages have this feature built-in, which is expected at this point.
Now, whenever a function is called, the program will usually pause the currently running program and implement the function. From there, the function will be read from top to bottom, and once the function has completed its task, the program will continue from where it had paused.
Should the function send back a value, that particular value will then be used where the function was originally called.
Read: What is Java Programming language?
How to write a Void function
OK, so writing a void function is super easy and can be done in a short amount of time. Bear in mind that this function does not return a value. Let’s look at a few examples that might give you an idea of what to do.
JavaScript Example
function helloFunction(){
alert("Hello World!");
}
helloFunction();
Python Example
def helloFunction():
print("Hello World")
helloFunction()
C++ Example
#include <iostream>
using namespace std;
void helloFunction(){
cout << "Hello World!";
}
int main(){
helloFunction();
return 0;
}
Read: What is the R programming language?
How to write Functions that require a value
If you are writing the same piece of code several times throughout your work, then void functions are perfect for that. However, these types of functions do not change, which doesn’t make them super useful. The best way to make void functions more beneficial is to increase what they can do by sending different values to the function.
Python Example
def helloFunction(newPhrase):
print(newPhrase)
helloFunction("Our new phrase")
JavaScript Example
function helloFunction(newPhrase){
alert(newPhrase);
}
helloFunction("Our new phrase");
C++ Example
#include <iostream>
using namespace std;
void helloFunction(string newPhrase){
cout << newPhrase;
}
int main(){
helloFunction("Our new Phrase");
return 0;
}
Read: Best Programming Principles & Guidelines all Programmers should follow.
How to write a Function that returns a value
The final aspect of this article, then, is how to write a function that will return a value. Whenever you want to alter data before using it, then this is the way to go in most situations.
Python Example
def addingFunction(a, b):
return a + b
print(addingFunction(2, 4))
JavaScript Example
function addingFunction(a, b){
return a + b;
}
alert(addingFunction(2, 4));
C++ Example
#include <iostream>
using namespace std;
int addingFunction(int a, int b){
return a + b;
}
int main(){
cout << addingFunction(2, 4) ;
return 0;
}
Read: The best projects for beginner Programmers.
Have fun testing the codes we’ve listed here. We hope they will prove useful in your work.